Table Of Content
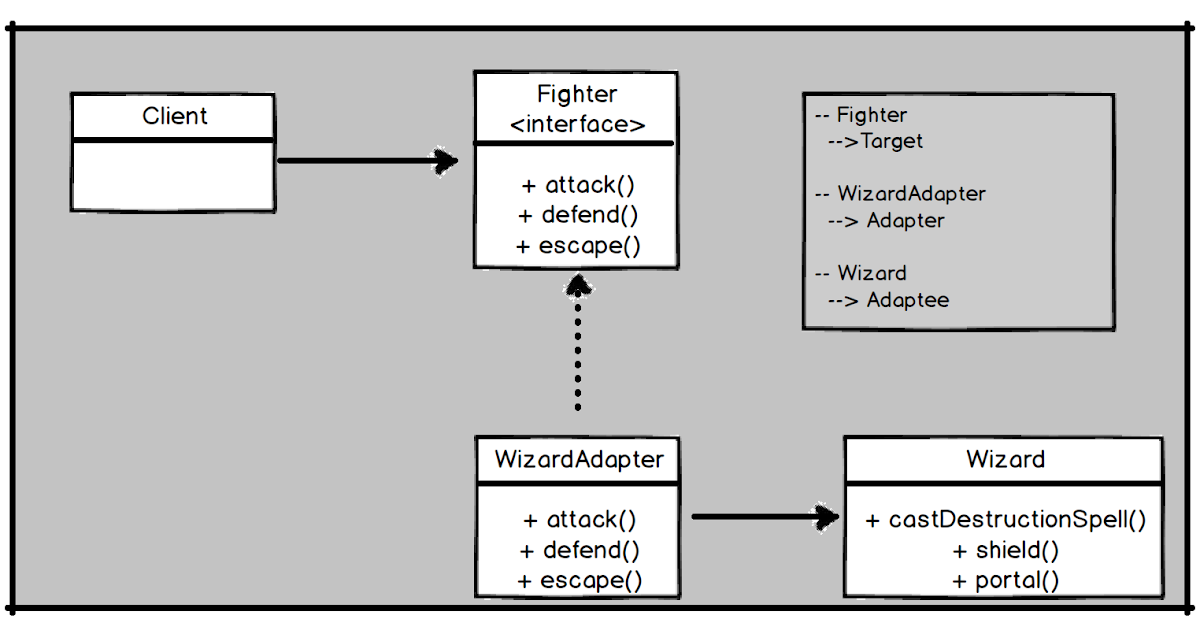
However, a constructor must always return new objects by definition. Subclasses can alter the class of objects being returned by the factory method. Now that we have super classes and sub-classes ready, we can write our factory class.
Factory Method in C++ / Design Patterns
Since every object consumes memory space that can be crucial for low memory devices, flyweight design pattern can be applied to reduce the load on memory by sharing objects. Bridge Method is a structural design pattern,it provide to design separate an object’s abstraction from its implementation so that the two can vary independently. Each concrete factory is responsible for creating an instance of a specific concrete product. In the example, INRFactory, USDFactory, and GBPFactory are concrete factory implementations. In Factory pattern, we create object without exposing the creation logic to the client and refer to newly created object using a common interface.
Pros and Cons of the Factory Pattern
AccountType consists of fixed values that have been predefined in the banking industry domain; therefore, it is a constant, and we use Enum for this data type to ensure integrity. Lets you separate algorithms from the objects on which they operate. Lets you save and restore the previous state of an object without revealing the details of its implementation. The pattern restricts direct communications between the objects and forces them to collaborate only via a mediator object.
2 Abstract Factory Method
The Factory Design Pattern is a creational pattern, meaning it is concerned with the process of object creation. By using this pattern, we can make the object creation process more flexible and modular, making it easier to change or add new object types in the future. If the factory is an interface - the subclasses must define their own factory methods for creating objects because there is no default implementation. Above class-diagram depicts a common scenario using an example of a car factory which is able to build 3 types of cars i.e. small, sedan and luxury. Building a car requires many steps from allocating accessories to final makeup. These steps can be written in programming as methods and should be called while creating an instance of a specific car type.
It reduces coupling between client code and object construction code and results in maintainable, scalable applications. Understanding when and how to apply the factory design pattern effectively can enhance your skills as a software developer and improve your applications. Factory method is a creational design pattern which solves the problem of creating product objects without specifying their concrete classes. In the factory design pattern, it says to define an interface (maybe a java interface or an abstract class) for creating the objects, and then the subclasses decide which classes to be instantiated.
Lets you produce families of related objects without specifying their concrete classes. The dependency injection pattern allows us to remove the hard-coded dependencies and make our application loosely-coupled, extendable, and maintainable. We can implement dependency injection in Java to move the dependency resolution from compile-time to runtime. The composite pattern is used when we have to represent a part-whole hierarchy.
Source code and design conformance, design pattern detection from source code by classification approach - ScienceDirect.com
Source code and design conformance, design pattern detection from source code by classification approach.
Posted: Fri, 11 Oct 2019 05:39:09 GMT [source]
Class Diagram
The factory pattern makes it possible by providing a static construction method which can be called with relevant parameters. The Factory Method defines a method, which should be used for creating objects instead of using a direct constructor call (new operator). Subclasses can override this method to change the class of objects that will be created.
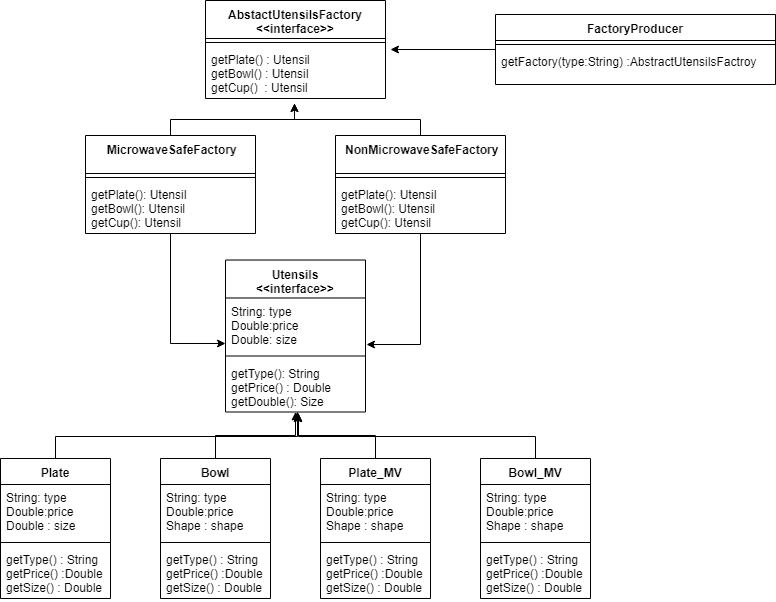
Disadvantages of the Factory Design Pattern in Java Design Patterns
So far we have design the classes need to be designed for making a CarFactory. You often experience this need when dealing with large, resource-intensive objects such as database connections, file systems, and network resources. Use the Factory Method when you want to save system resources by reusing existing objects instead of rebuilding them each time.
They offer clever solutions to some common problems and encourage developers and entire teams to do architecture design first, programming second. This almost always leads to a higher-quality code rather than jumping right into programming. Then, using a SpaceshipFactory as our communication point with these, we'll instantiate objects of Spaceship type, though, implemented as concrete classes. This logic will stay hidden from the end user, as we'll be specifying which implementation we want through the argument passed to the SpaceshipFactory method used for instantiation. Instead of hard-coding object creation into the createSpaceship() method with new operators, we'll create a Spaceship interface and implement it through a couple of different concrete classes. If the factory is a class - the subclasses can use existing implementation or optionally override factory methods.
Notification Interface as a base interface for creating/implementing different object classes. This approach separates the object creation from the implementation, which promotes loose coupling and thus easier maintenance and upgrades. For this reason, design patterns have become the de facto standard in the programming industry since their initial use a few decades ago due to their ability to solve many of these problems.
Here every catch block is kind of a processor to process that particular exception. So when an exception occurs in the try block, it’s sent to the first catch block to process. If the catch block is not able to process it, it forwards the request to the next Object in the chain (i.e., the next catch block). If even the last catch block is not able to process it, the exception is thrown outside of the chain to the calling program. Template Method is a Behavioral Design Pattern, it defines the skeleton of an algorithm in a method but lets subclasses alter some steps of that algorithm without changing its structure. Then we have the static method getCoin to create coin objects encapsulated in the factory class CoinFactory.
All the code additions are similar to those done for the Petrol.java file. In our example, we’ll create a food ordering system that allows customers to order different types of dishes, such as pizza and sushi, using the Factory Pattern. The interpreter pattern is used to define a grammatical representation of a language and provides an interpreter to deal with this grammar. The proxy pattern provides a placeholder for another Object to control access to it. This pattern is used when we want to provide controlled access to functionality.
This pattern treats both individual objects and compositions of objects it allow clients to work with complex structures of objects as if they were individual objects. In this article we talked about the factory design pattern and it’s implementation using Java. We talked about it’s benefits and when we should use this design pattern in our application. To understand factory design pattern more easily, let’s take an example where we are building a banking application. The initial release is focused on providing a saving account for the customers so most of our code and focus will be on the SavingAccount class. But we have the plan to launch other type of accounts for the customers.
The Factory Design Pattern is a promising candidate because its purpose is to manage the creation of objects as required. Defines the skeleton of an algorithm in the superclass but lets subclasses override specific steps of the algorithm without changing its structure. Lets you define a family of algorithms, put each of them into a separate class, and make their objects interchangeable.
Your app should have round buttons, but the framework only provides square ones. You extend the standard Button class with a glorious RoundButton subclass. But now you need to tell the main UIFramework class to use the new button subclass instead of a default one. The first version of your app can only handle transportation by trucks, so the bulk of your code lives inside the Truck class. Let’s say we have two sub-classes PC and Server with below implementation. To solve the problem mentioned above, we can apply the Factory Design Pattern.
No comments:
Post a Comment